Basic Input Output
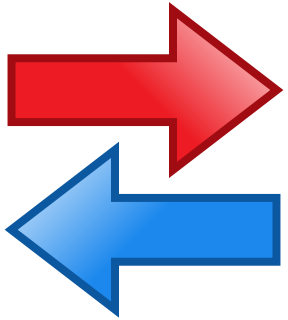
Basic input/output:- Input refers to accepting data from outside the program to the program while output refers to presenting the programmed data as a result of programming. Every program performs three main functions accepting data from user, processing it and producing the output. In C language there are several input and output functions.These functions are collectively found in "IO.h" and such IO function together form a library name "stdio.h" .User will require all such library function. These library functions are classified into three broad categories: 1. Console I/O functions:- Console I/O refers to the operation that occur at the keyboard and the screen of your computer . Different streams are used to represent different kinds of data flow. In C there are 3 streams associated with console I/O operations. These are:- Stdin:- It is the stream which supplies the data from input sources(i.e keyboard) to progr...